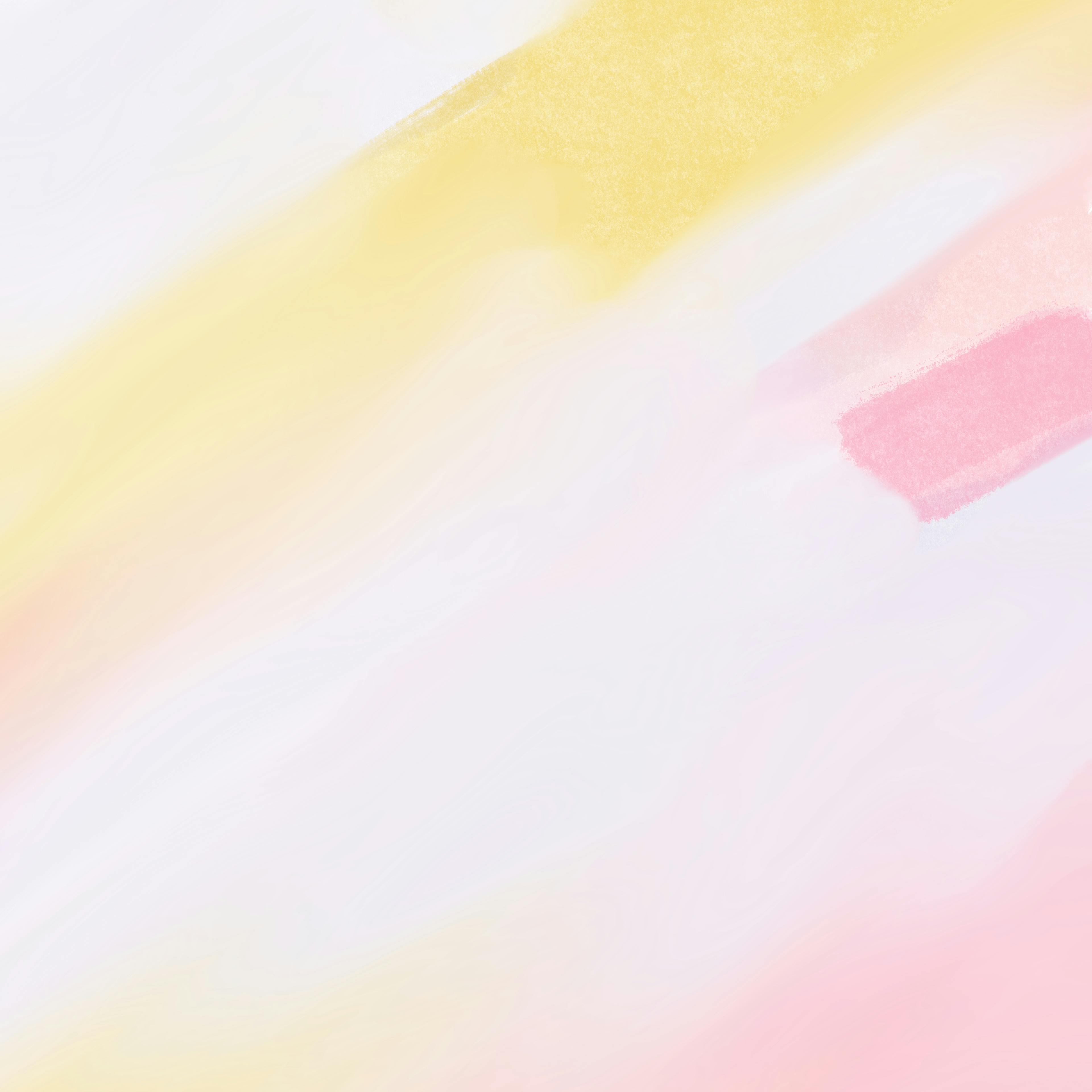
Garden scene loading...
Garden scene loading...
Page coming soon
Cinderella's still sweeping chimneys and singing to woodland animals: in other words, our web dev fairy godmother hasn't arrived to work her magic on this page just yet. 😉
Why don't you use one of the links in the menu above to choose another adventure for now?